Tutorial: Getting Familiar With Nuxt OG Image
Get started with the module by setting up your first og:image on your home page.
This is a three-part tutorial to getting familiar with the module. It's recommended to follow when you use the module for the first time.
Part 1: Using An OG Image
To start with, we just want to be able to see the module generating an image for us, any image and playing around with different options we can provide to change it.
Make sure you have the module installed and Nuxt DevTools enabled before starting.
1. Define an OG Image
Firstly, we're going to use the server-only composable defineOgImageComponent
to define the og:image
for our home page.
<script lang="ts" setup>
defineOgImageComponent('NuxtSeo')
</script>
This will use the default template NuxtSeo.
2. View your og:image
Visit the home page in your browser and open up the Nuxt DevTools (Shift
+ Alt
+ D
).
Once you're in the Nuxt DevTools, you can navigate to the OG Image tab by opening the command palette (Ctrl
+ K
) and typing og
.
You should now see a preview of your OG Image.

Part 2: Customising NuxtSeo Template
Now that we can see our OG Image, we're going to customize it by modifying the props we pass to the defineOgImageComponent
composable.
Feel free to pass in any props you like, but for this example we're going to use the following:
<script lang="ts" setup>
defineOgImageComponent('NuxtSeo', {
title: 'Hello OG Image ๐',
description: 'Look what at me in dark mode',
theme: '#ff0000',
colorMode: 'dark',
})
</script>
The playground has full HMR, you should see an image that comes through like this straight away.

Congrats, you've set up and customized your first og:image
!
Going further, we can even try using one of the other community templates available. For the full list, checkout the Community
tab within
Nuxt DevTools.
<script lang="ts" setup>
defineOgImageComponent('Nuxt', {
headline: 'Greetings',
title: 'Hello OG Image ๐',
description: 'Look what at me using the Nuxt template',
})
</script>
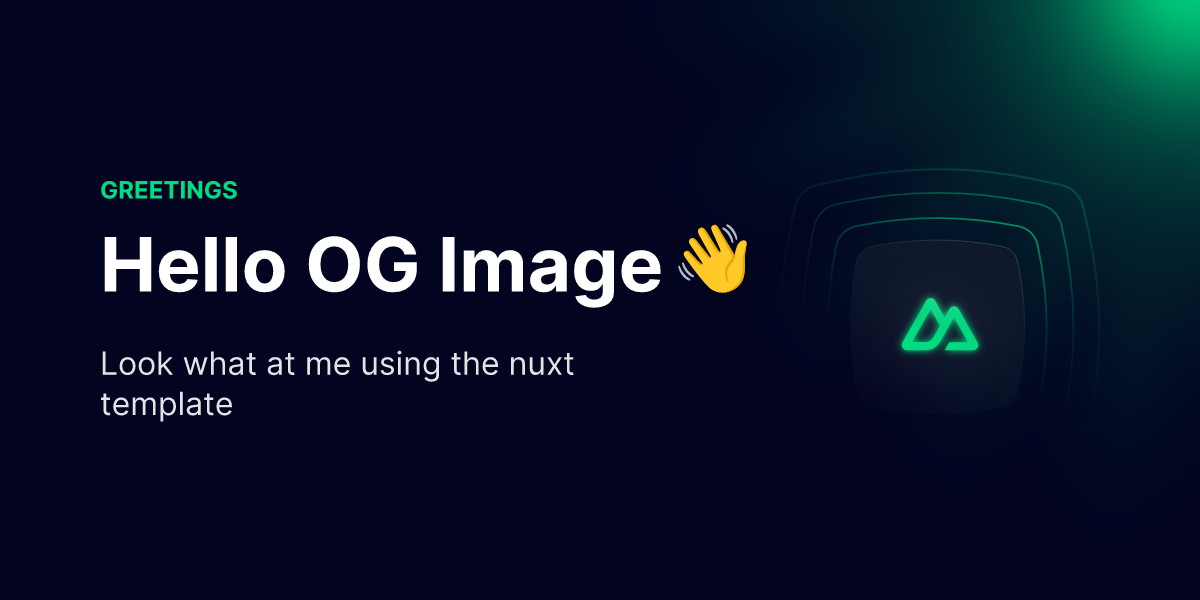
You can see all the supported props on the NuxtSeo Template documentation. It's also worth checking out the defineOgImage API.
Part 3: Creating Your Own Template
Using the community templates is a fun way to experiment with the OG Image template you want to use. However, they will always be limited in what you can do with them.
For this reason it's recommended to copy+paste the template you want to use into your project and customize it from there.
You can find the template source code within the Community
tab of Nuxt DevTools or on GitHub.
1. Create your template component
We're going to start with the SimpleBlog template as a quick way to get started.
Let's copy this template into our project at ./components/OgImage/BlogPost.vue
and remove some of the boilerplate.
Anything components you add to a OgImage
folder will be automatically registered as a template for you.
<script setup lang="ts">
withDefaults(defineProps<{
title?: string
}>(), {
title: 'title',
})
</script>
<template>
<div class="h-full w-full flex items-start justify-start border-solid border-blue-500 border-[12px] bg-gray-50">
<div class="flex items-start justify-start h-full">
<div class="flex flex-col justify-between w-full h-full">
<h1 class="text-[80px] p-20 font-black text-left">
{{ title }}
</h1>
<p class="text-2xl pb-10 px-20 font-bold mb-0">
mycoolsite.com
</p>
</div>
</div>
</div>
</template>
2. Use the new template
Now that you have your template, you can use it in for your home page.
<script lang="ts" setup>
defineOgImageComponent('BlogPost', {
title: 'Is this thing on?'
})
</script>
Check your Nuxt DevTools to see the new template in action.
You may notice that the Tailwind classes just work, even if you're not using the Tailwind module.
In fact UnoCSS and Tailwind classes are supported out of the box and will be merged with your default theme config. You can learn more about this in the Styling guide.
3. Customize your template
Now that you have your template, you can start customizing it.
Any props you pass to the defineOgImageComponent
composable will be available in the component.
With this in mind let's add a new prop to change the border color borderColor
.
It's recommended to always use a withDefaults
wrapper around your props to provide default values. This allows
you to preview the template when you're not passing any props.
<script setup lang="ts">
withDefaults(defineProps<{
title?: string
borderColor?: string
}>(), {
title: 'title',
borderColor: 'blue-500'
})
</script>
<template>
<div :class="[`border-${borderColor}`]" class="h-full w-full flex items-start justify-start border-solid border-[12px] bg-gray-50">
<div class="flex items-start justify-start h-full">
<div class="flex flex-col justify-between w-full h-full">
<h1 class="text-[80px] p-20 font-black text-left">
{{ title }}
</h1>
<p class="text-2xl pb-10 px-20 font-bold mb-0">
mycoolsite.com
</p>
</div>
</div>
</div>
</template>
Now let's customize the border to be a light green instead.
<script lang="ts" setup>
defineOgImageComponent('BlogPost', {
title: 'Is this thing on?',
borderColor: 'green-300',
})
</script>
Within the playground, you should now see the background color change to green.
Conclusion
Thanks for following along! You know have a basic understanding of how to use the module.
It's recommended to look through the rest of the documentation to get a full understanding of what's possible.
If you have any questions, feel free to reach out on Discord or GitHub.
๐ Support My Work
This module has taken me over 250 hours to build. If amazing SEO DX for Nuxt is important to you, consider supporting my work on Nuxt SEO.
Thank you to all my existing sponsors.